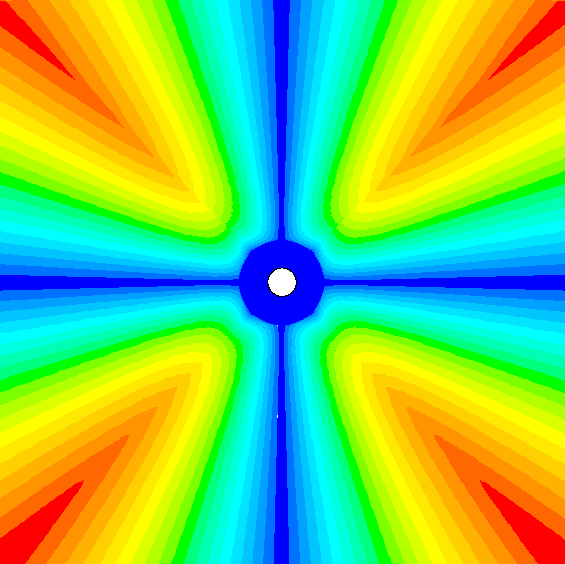 STAR-CCM+ example Java codes |
/* Store common variables for mesh generation, boundary conditions, material
properties, solver settings, post-processing and report generation in separate
file. The constants and variables are stored in file simVars.java and the main
function and methods are stored in file named solverSettingsSTAR.java. The two
files need to be compiled together: javac solverSettingsSTAR.java simVars.java
*/ |
Contents of simVars.java
public final class simVars {
private simVars() {
// Restrict instantiation
}
public static final double ref_den = 1.225;
public static final double Tref = 300;
} |
Contents of solverSettingsSTAR.javapublic class solverSettingsSTAR {
public static void main(String[] args) {
System.out.println(simVars.ref_den);
}
} |
Methods and Classes: uncomment/comment as needed.import java.util.*;
import star.common.*;
import star.base.neo.*;
import star.motion.*;
public class starMacroTemplate extends StarMacro {
public void execute() {
importCad_Mesh();
generateSrfMesh();
generateVolMesh();
defineMatProps();
defineBndConds();
solverSettings();
defineMonitors();
defineReports();
generateReports();
defineScenes();
saveReptPlots();
}
private void importCad_Mesh() {
...
}
private void generateSrfMesh() {
...
}
private void generateVolMesh() {
...
}
private void defineMatProps() {
...
}
private void defineBndConds()() {
...
} |
private void solverSettings() {
Simulation sim_1 = getActiveSimulation();
for (int i=1; i<=3; i++) {
sim_1.saveState("Case_" + i + ".sim");
...
StepStoppingCriterion stopCrit = (StepStoppingCriterion) sim_1.getSolverStoppingCriterionManager()
.getSolverStoppingCriterion("Maximum Steps");
//Get max stopping criteria number and add additional iteration steps
IntegerValue iter_count = stopCrit.getMaximumNumberStepsObject();
double j = iter_count.getQuantity().getValue();
iter_count.getQuantity().setValue(j);
sim_1.getSimulationIterator().run();
}
// Save final sim file and exit
sim_1.saveState("Case_" + i + "_Full.sim");
}
} |
private void defineMonitors() {
...
} |
private void defineReports() {
...
} |
private void defineScenes() {
...
} |
private void generateReports() {
...
} |
private void saveReptPlots() {
...
} |